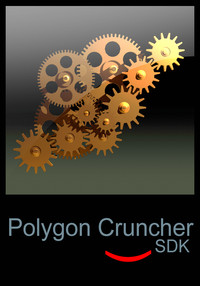
Polygon Cruncher SDK
Overview
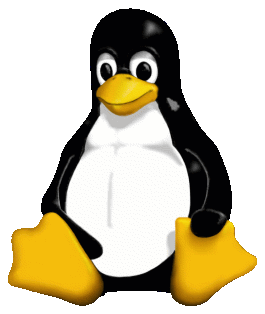
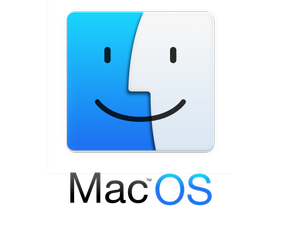
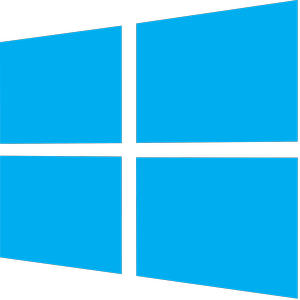
Polygon Cruncher SDK is a robust, powerful fast and multithreaded 3D C++ library for Windows, macOS & Linux.
It includes a 3D scene graph with powerful features dedicated to 3D I/O and mesh optimization.
It also many capabilities, such:
- Polygon Cruncher optimization multithreaded algorithm, LOD generation and batch support.
Giving a ratio, Polygon Cruncher will decimate your 3D meshes up to this level of simplification.
- MagicCruncher algorithm which will find the optimal ratio for you, which is the value that gives the best visual results.
With MagicCruncher, decimation can be automated in a 3D pipeline or for providing web services.
- Read and write capabilities for most common 3D file formats, with the ability to set the relevant options for each format - for embedding textures for example.
Support Fbx, Sketchup, GLB/GLTF, Blender, Cinema 4D, 3ds, lxo, collada, wavefront, vrml... using few line of codes.
- You can also register your own 3D parser to integrate it fully to the SDK an make it simple to support your own data.
- A complete scene graph with simple access to scene hierarchy, scene nodes, objects, and transformations.
- Instantiable N-Gon objects with support for UVs, vertex colors, normals information.
You can attach any type of additional data to points, faces. This data follows the object workflow and can be retrieved at the end of a process.
- A class of dynamic multi-resolution objects that are meshes for which you can modify the level of detail in real time
These objects are calculated after optimizing the 3D scene supplied. They can also be built from previously calculated and stored data.
They can also be streamable, in which case the 3D object is built as the data is received.
- Support for standard materials, PBR material and material maps.
- Advanced set of 3D related methods for mesh cleaning, triangulation, confused point welding, mesh merging using different criteria such materials, size or anything else...
- Fast and powerful ray casting and closest face finding methods.
- The SDK is very efficient in terms of memory requirements. You can plug your own memory allocation callbacks.
The SDK can handle huge 3D objects in terms of data volume and processing capacity.
- All needed classes / functions for file access, handling path, unicode / utf8 strings, sockets, threads compatible with different OS.
- Optional support for JT files through Siemens JT SDK. Check out here JT capabilities.
The SDK is available as a C++ library:
- for Windows issued from Visual Studio 2022 / 64 bits.
- for MacOS issued from XCode (Universal binaries).
- for Linux (Centos / gcc 4)
- A .Net wrapper
The API is simple to use and implement and you can add decimation capabilities quickly to your software, your 3D pipeline, your specific process.
The application domain covers any 3D fields where LOD models are needed: video games, CAD, real time visualization, 3D scanning, 3D modeling, 3d photogrametry, mesh reconstruction from point clouds...
Polygon Cruncher SDK provides a robust, proven technology developed since 1999. It is used by many companies (Autodesk, Anark, 2020, ka-ra...) in different contexts: architectural software, 3d software, CAO & FAO.
Mootools provides a close support during the integration phase, making the implementation process fast and easy.
Mootools gives access to its software (3DBrowser Pro / Polygon Cruncher) which include the SDK and expose its features. This make easier to validate each steps of the implementation and check out that data are correctly retrieved.
SDK Optimization Pipeline
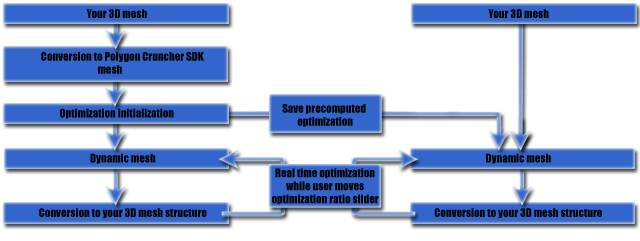
Polygon Cruncher SDK pipeline
This diagram proposes 2 different integration possibilities: one with user interaction, the other using pre-calculated optimization.
- The left side exposes an optimization pipeline in which the user is associated to the optimization choice.
The user makes the choices for keeping textures or not, protect seams or normals selecting the most appropriate settings for optimizing its 3D assets.
Once optimization is processed and decimation performed, the SDK provides a multi-resolution dynamic mesh.
Number of point and faces can be modified in real time thanks to multiresolution mesh. This is a way to give an immediate feedback to the user which can choose the appropriate LOD (level of details) in real time.
Polygon Cruncher Polygon Cruncher software or ProOptimizer (Autodesk 3ds Max plug-in based on an older version of the SDK) is a good demonstration of such integration of the SDK. - The right side shows a different approach. In this case, the caller provides to the SDK a 3D mesh as well as precomputed optimization data.
This data might have been created previously using the most appropriate optimization settings for the mesh.
With that information, the optimization step is removed and the SDK runs in real time: dynamic multi resolution mesh is generated immediately and the LOD can be adjusted in real time.
This might be an interesting schema: avoid the data calculation step and directly get a dynamic multi resolution mesh using pre-optimized data for real time visualization context.
Of course, this is just one integration proposal among many. Mootools will work with you to find the one that best fits your 3D workflow.
Optimization Implementation
The implementation is straightfoward. The entry point is converting your 3D data information to / from Polygon Cruncher SDK 3D data structure.
The SDK offers N-gons objects, which are polygonal mesh with N-sided faces.
You can integrate your specific data, so that it flows seamlessly into the process.
The simplification algorithm receives in input your N-sided polygonal meshes. It returns triangular meshes with texture, vertex colors and normal maps, depending on the information provided.
Initialization
Depending on your needs, you can provide a single mesh or a whole scene to the optimizer. Each mesh may only contain the polygonal information or a more complex data set which can include:
- One or more UV texture channel.
- One or more VC vertex color channel.
- An user normal channel.
- A selection information which give a control on the optimizable part of the geometry.
- Optionnaly some additional data provided by you.
You have then to define the optimization mode. You'll retrieve all Polygon Cruncher optimization modes available through the API such seams preservation (preserve / protect / exclude borders modes, 3D point lock, generate compact faces...
Optimization computation
Then you trigger optimization and the SDK performs a first initialization step. You then have multiple choice:- Static optimization: you'd like the scene to take on different levels of detail. You can output LOD from this initialization step without the need of multi-resolution object.
- Dynamic optimation: you can get a multiresolution mesh from the pre-calculation giving the ability to optimize in real time in the following step (Real time optimization feedback loop).
- If your process does not include any user interaction, MagicCruncher is the winning choice, as arbitrarily determining an optimization ratio can be a bad idea. MagicCruncher will compute automatically the optimal ratio which is the simplification level that gives the best balance between high ratio and perfect visual result.
For example, in architectural context scenes might contains walls, floors and various type of objects that involve low level of optimization (a 6 faces cube cannot be simplified in any way!).
MagicCruncher will use different levels to optimize objects that cannot be optimized as much, and those that can be considerably lightened.
Real time optimization feedback loop and termination
If the process includes user feedback, you usually gives him a chance to visually select the appropriate optimization ratio through a slider and a interactive feedback.
As the initialization phase has been computed before, the process is now very fast and perfect for providing a real time feedback.
On the implementation side, this feedback is obtained easily by converting back the SDK structure into your own 3D structure.
The process might also involves to provide a display of the 3D content that depends on different criteria (rendering time, details depending on the camera distance or anything else).
Saving multi resolution mesh
The dynamic mesh can be saved to the disk, so it can be reinitiallized and recreated on demand in a blink.
Saving pre-computed data avoid the initialization step but keep the ability to modify the level of details (LOD) on demand.
It's possible to save complete optimized data set including all channels (textures, vertex colors, normals) and then to use only a part of this data afterward (ie only textures).
The dynamic mesh can also be a 3D streamable mesh. The multiresolution data can be streamed over the network. On the receiving side, the object is reconstructed as data is received.
DirectX 9 sample
Download sample applicationThis sample application shows some features of the SDK used in a DirectX 9 context. It's an old compilation (sorry for that) from the provided sample available for both x32 and x64 bits Windows platforms.
You need to have DirectX9 setup on your computer in order to run this sample.
The sample load a default mesh and optimize it.
Load an object (O key)
You can load wavefront obj, directx or pOpt files.
If you load Obj file or DirectX file, the mesh is loaded and optimized. Then it is converted to a dynamic mesh. This demonstrates the left part of the below pipeline.
If you load a popt file, the sample loads a precomputed optimized mesh. There is no optimization step and the dynamic mesh is directly available. This demonstrates the right part of the below pipeline.
Save a pre-computed optimized mesh (S key)
This option optimize the loaded mesh and save the optimization to a popt file located in the mesh directory.
Example: if the mesh is furniture.x, then a furniture.popt is saved in furniture directory.
Once computed, you can load this file using O key. Just select the popt file.
This popt file saves the optimization as a dynamic mesh. When loaded it removed the heavy computation process step and allows the user to get the dynamic mesh directly with the appropriate optimization settings.